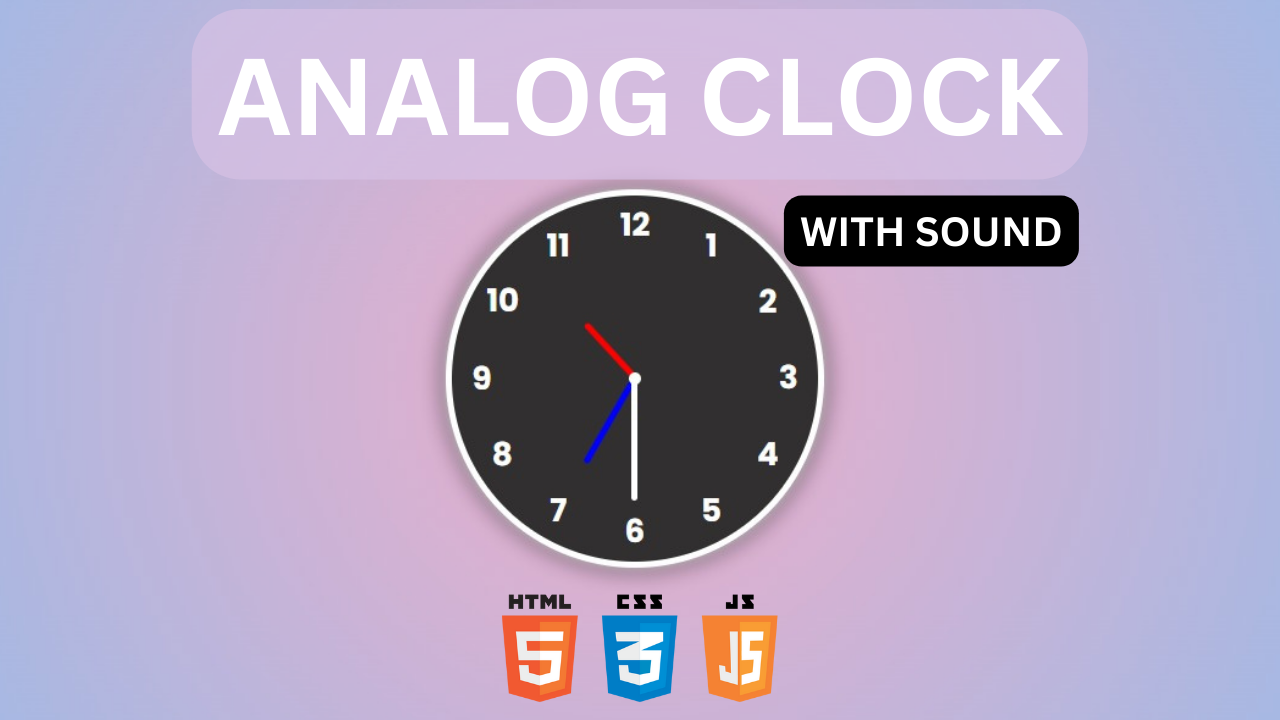
Introduction
Have you ever wanted to create an analog clock with sound? In this post, we will learn how to design and implement an analog clock with sound using HTML, CSS, and JavaScript.
So, let’s get started!
1. What is an Analog Clock?
An analog clock is a traditional timekeeping device that displays time through the position of hands on a circular dial. It consists of an hour hand, a minute hand, and sometimes a second hand. In this tutorial, we’ll recreate this classic timepiece using HTML, CSS, and JavaScript.
2. Setting Up the HTML Structure
To start, we’ll create the basic HTML structure for our analog clock.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Analog Clock in HTML - CSS - JS</title> <!-- Custom CSS --> <link rel="stylesheet" href="style.css"> </head> <body> <!-- Clock --> <div class="clock"> <div class="needle hr" style="--h:60px; --clr:red"><span></span></div> <div class="needle mn" style="--h:80px; --clr:blue"><span></span></div> <div class="needle ss" style="--h:100px; --clr:white"><span></span></div> <ul> <li style="--i:1"><span>1</span></li> <li style="--i:2"><span>2</span></li> <li style="--i:3"><span>3</span></li> <li style="--i:4"><span>4</span></li> <li style="--i:5"><span>5</span></li> <li style="--i:6"><span>6</span></li> <li style="--i:7"><span>7</span></li> <li style="--i:8"><span>8</span></li> <li style="--i:9"><span>9</span></li> <li style="--i:10"><span>10</span></li> <li style="--i:11"><span>11</span></li> <li style="--i:12"><span>12</span></li> </ul> </div> <!-- Custom JS --> <script src="script.js"></script> </body> </html>
3. Styling the Analog Clock with CSS
Next, we’ll style our analog clock using CSS. We’ll also import the Google Fonts “Poppins” so that the fonts look nicer.
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;700&display=swap'); *{ margin: 0; padding: 0; } body{ font-family: 'Poppins', sans-serif; background: rgb(238,174,202); background: radial-gradient(circle, rgba(238,174,202,1) 0%, rgba(148,187,233,1) 100%); position: relative; display: flex; justify-content: center; align-items: center; height: 100vh; } .clock{ background-color: rgb(49,47,47); width: 300px; height: 300px; border-radius: 50%; color: #fff; border: 5px solid #fff; box-shadow: 0px 0px 10px rgba(255,255,255,0.7), 0px 0px 20px rgba(0,0,0,0.7); font-size: 26px; font-weight: bold; display: flex; justify-content: center; align-items: center; position: relative; } .clock ul li{ list-style: none; position: absolute; text-align: center; transform: rotate(calc(30deg * var(--i))); inset: 5px; } .clock ul li span{ transform: rotate(calc(-30deg * var(--i))); display: inline-block; } .clock:after{ content: ''; width: 10px; height: 10px; background-color: #fff; border-radius: 50%; position: absolute; } .needle{ display: flex; justify-content: center; align-items: flex-end; } .needle span{ width: 5px; height: var(--h); background-color: var(--clr); position: absolute; border-radius: 5px; }
4. Adding Functionality with JavaScript
Now it’s time to make our analog clock functional using JavaScript. We’ll use JavaScript to update the position of the clock needles based on the current time. To make our analog clock more engaging, we can add sound effects to indicate the ticking of the clock.
function show_clock(){ let h = document.getElementsByClassName('hr')[0]; let m = document.getElementsByClassName('mn')[0]; let s = document.getElementsByClassName('ss')[0]; let date = new Date(); let hours = date.getHours(); let minutes = date.getMinutes(); let seconds = date.getSeconds(); h.style.transform = `rotate(${30 * hours + minutes/2}deg)`; m.style.transform = `rotate(${6 * minutes}deg)`; s.style.transform = `rotate(${6 * seconds}deg)`; let sound = new Audio('sound.mp3'); sound.play(); } setInterval(show_clock, 1000);
5. Testing and Troubleshooting
Make sure the clock needles move correctly and the sound effects play as expected. If you encounter any issues, check your code for errors and also check errors in inspect elements of the browser.
6. Conclusion
Congratulations! You have successfully learned how to create an analog clock with sound using HTML, CSS, and JavaScript.
FAQs
Q1: Can I customize the appearance of the analog clock?
Yes, you can customize the analog clock’s appearance by modifying the CSS code. Adjust the sizes, colors, and positions to match your website’s design.
Q2: How can I change the ticking sound of the clock?
You can replace the “sound.mp3” file in the code with your desired sound file. Make sure to update the file name and extension accordingly.
Q3: Does the analog clock work on all browsers?
Yes, the analog clock should work on most modern web browsers, including Chrome, Firefox, Safari, and Edge.
Q4: Can I add additional functionality to the analog clock?
Absolutely! You can extend the functionality of the analog clock by adding features such as alarm settings, different time zones, or additional animations.
Q5: Is it possible to embed the analog clock on multiple web pages?
Yes, you can reuse the analog clock’s HTML, CSS, and JavaScript code on multiple web pages by linking to the same files or copying the code snippet.
I wonder about your fixwebissues.com very excellent work n perfect task I hope u do so..alway God bless your team.
TQ so much,.
Regard
Syed Jaffer
by SAMA Computers 🖥️.