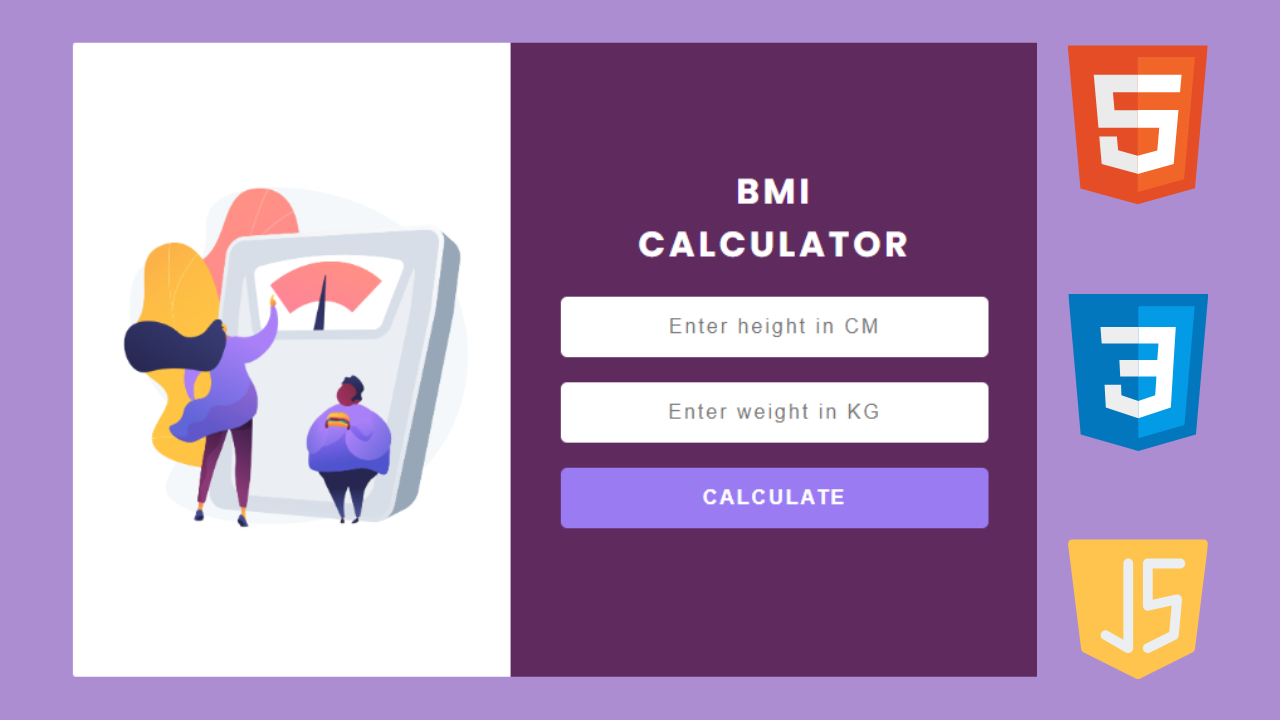
Build BMI Calculator in HTML, CSS, and JavaScript
1- Introduction
BMI Calculator, Managing our body mass index (BMI) is essential to maintaining a healthy lifestyle in today’s health-conscious world. By calculating our BMI, we can determine if we are overweight, normal weight, underweight, or obese. In this article, we will walk you through the process of creating a BMI calculator using HTML, CSS, and JavaScript.
2- What is BMI?
BMI stands for Body Mass Index. It is based on a person’s height and weight. The BMI formula is as follows:
BMI = weight (kg) / (height (m))^2
The resulting score indicates whether a person has a healthy or unhealthy weight in relation to their height. BMI is a widely used metric to check whether a person is underweight, normal weight, overweight, or obese.
3- Why Use a BMI Calculator?
A BMI calculator is a useful tool that calculates the BMI based on the user’s weight and height inputs. It removes the need for manual calculations and provides fast and accurate results.
4- Setting Up the HTML Structure
To create a BMI calculator, we first need to set up the HTML structure. Begin by opening a new HTML file in your favorite text editor. Here’s the code of a basic HTML structure:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>BMI - in HTML, CSS and JS</title> <link rel="stylesheet" href="style.css"> </head> <body> <div class="container"> <div class="image-container"> <img src="bmi.jpg" alt="BMI bg"> </div> <div class="form-container"> <h1>BMI</h1> <h2>Calculator</h2> <div class="form"> <form action=""> <input type="text" class="height" placeholder="Enter height in CM"> <input type="text" class="weight" placeholder="Enter weight in KG"> <input type="submit" value="Calculate"> </form> </div> </div> <div class="popup"> <div class="bmi-score"></div> <div class="bmi-text"></div> <button class="close-btn">Close</button> </div> </div> </body> </html> <script src="script.js"></script>
5- Styling the BMI Calculator with CSS
Now that we have set up the HTML structure, let’s style the BMI calculator using custom CSS. Create a new file called “style.css” and link it to your HTML file using the `<link>` tag in the `<head>` section. Here’s the code of CSS styles for the BMI calculator:
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;700&display=swap'); *{ margin:0; padding:0; } body{ display: flex; justify-content: center; align-items: center; background: #f490f1; background: linear-gradient(270deg, #f490f1 0%, #a08dce 100%); font-family: 'Poppins', sans-serif; height: 100vh; } .container{ max-width: 768px; display: flex; justify-content: center; align-items: center; background-color: #fff; box-shadow: 0px 0px 10px rgba(0,0,0,0.1); border-radius: 5px; } .image-container{ background-color: #fff; } .image-container img{ width: 100%; } .form-container{ background: #5f2a5e; padding: 100px 40px; text-align: center; border-radius: 0px 5px 5px 0px; } .form-container h1, .form-container h2{ font-size: 28px; font-weight: bold; text-transform: uppercase; color: #fff; letter-spacing: 3px; } .form-container .form{ width: 340px; } .form-container input{ font-size: 16px; display: block; padding: 15px 0px; border-radius: 5px; border: none; width: 100%; margin: 20px 0px; text-align: center; letter-spacing: 2px; } .form-container input:focus{ outline: none; } .form-container input[type="submit"]{ background-color: #9a7bf2; font-size: 16px; font-weight: bold; text-transform: uppercase; color: #fff; cursor: pointer; transition: .5s all; } .form-container input[type="submit"]:hover{ background-color: #fec045; } .popup{ position: absolute; background: #312222; padding: 50px 100px; border-radius: 5px; display: none; text-align: center; box-shadow: 0px 0px 70px -20px rgba(0,0,0,0.5); } .bmi-score{ width: 100px; height: 100px; background: #5254ce; border-radius: 50%; display: flex; justify-content: center; align-items: center; border: 5px solid #fff; font-size: 22px; color: #fff; font-weight: bold; margin: 0 auto; } .bmi-text{ margin: 20px 0px; background: #fff; padding: 10px 10px; border-radius: 5px; font-weight: bold; } .close-btn{ padding: 10px 30px; font-size: 16px; background: red; border: none; border-radius: 5px; color: #fff; font-weight: bold; cursor: pointer; } @media screen and (max-width: 768px) { .form-container{ width: 100%; } .image-container{ display: none; } }
6- Implementing the JavaScript Functionality
To add our BMI calculator functionality, we need to add JavaScript code. Create a new file called “script.js” and include it in the HTML file using the `<script>` tag before the closing `</body>` tag. Here’s the code of JavaScript code for the BMI calculator:
const form = document.querySelector('form'); const popup = document.querySelector('.popup'); const close = document.querySelector('.close-btn'); const height_input = document.querySelector('.height'); const weight_input = document.querySelector('.weight'); //Click the calculate button form.addEventListener('submit', function (e) { e.preventDefault(); let height = parseInt(height_input.value); let weight = parseInt(weight_input.value); const bmi_score = document.querySelector('.bmi-score'); const bmi_text = document.querySelector('.bmi-text'); let text; if (height === '' || height < 0 || isNaN(height)) { alert("Please enter a valid height"); height_input.focus(); } else if (weight === '' || weight < 0 || isNaN(weight)) { alert("Please enter a valid weight"); weight_input.focus(); } else { const bmi = (weight / ((height * height) / 10000)).toFixed(2); //show the result if (bmi < 25) { text = 'You are underweight'; } else if (bmi >= 25 && bmi < 30) { text = 'You are a healthy weight'; } else { text = 'You are overweight'; } //Show the popup popup.style.display = 'block'; //Display the bmi score bmi_score.innerHTML = bmi; //Display the bmi text bmi_text.innerHTML = text; //Clear the value of input fields height_input.value = ''; weight_input.value = ''; } }); //Close the popup close.addEventListener('click', function (e) { popup.style.display = 'none'; });
7- Conclusion
In the above article, we learned how to build a BMI calculator using HTML, CSS, and JavaScript.
8- FAQs
Q1: Can I customize the appearance of the BMI calculator?
Yes, you can customize the BMI calculator appearance by modifying the CSS code. Adjust the sizes, colors, and positions to match your website’s design.
Q2: How can I change the Image of the BMI calculator?
You can replace the “bmi.jpg” image file in the code with your desired image file. Make sure to update the file name and extension accordingly.
Q3: Does the BMI calculator work on all browsers?
Yes, the BMI calculator should work on all web browsers, including Chrome, Firefox, Safari, and Edge.
Q4: Can I add additional functionality to the BMI calculator?
Absolutely! You can extend the functionality of the BMI calculator by adding features such as Login, Registration, User Dashboard, and Animations.
Q5: Is the BMI calculator will work on Mobile?
Yes, the BMI calculator is fully responsive and it will work on any device like Mobile, Tablet, and Desktop.